Introduction
I have many campaign but some of them have a limit of usage per account. How to manage it?
Object you need:
Campaign
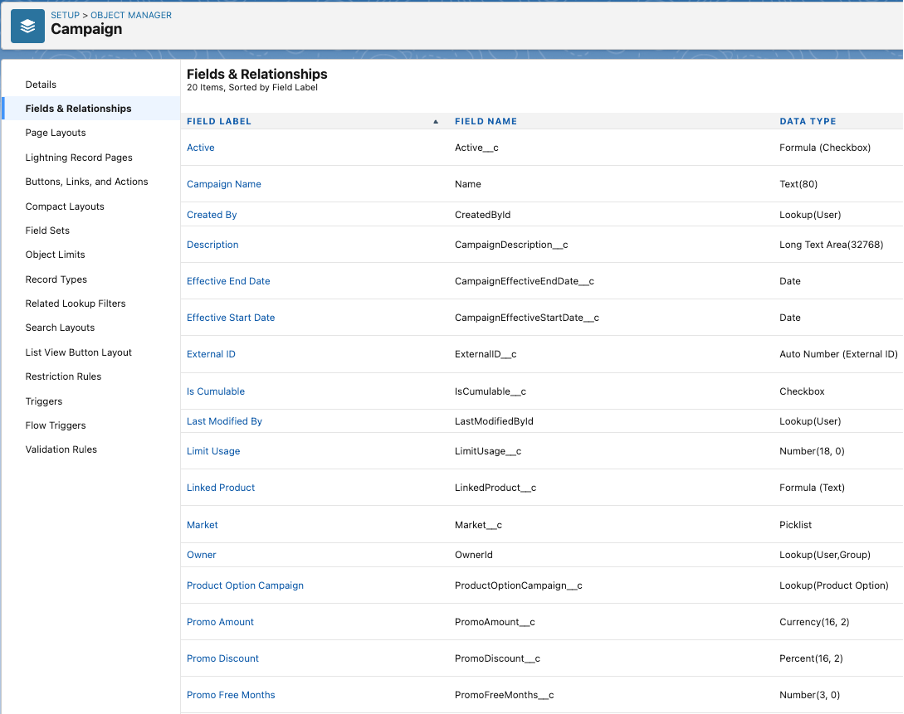

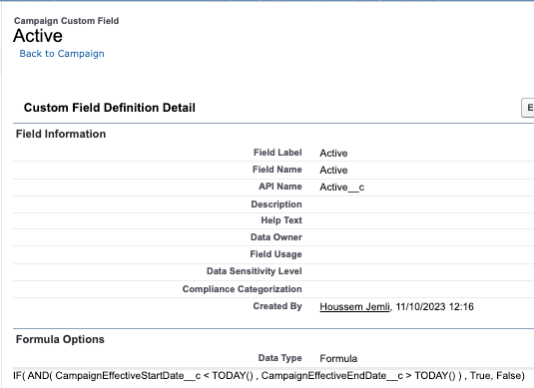
Coupon
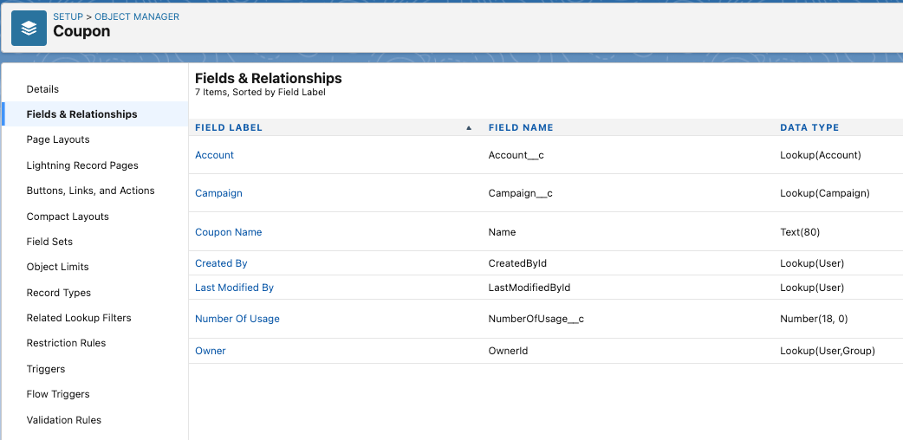
Field you need in existing object:
In quote

Flow you need:
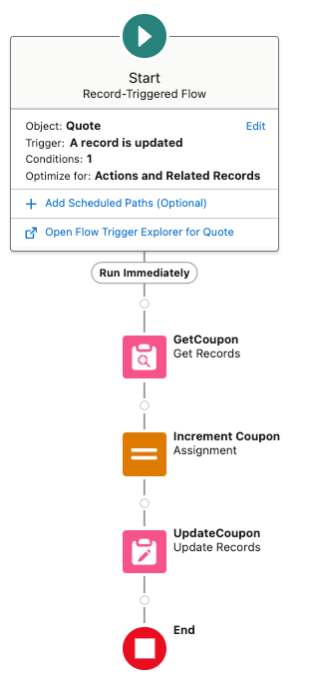
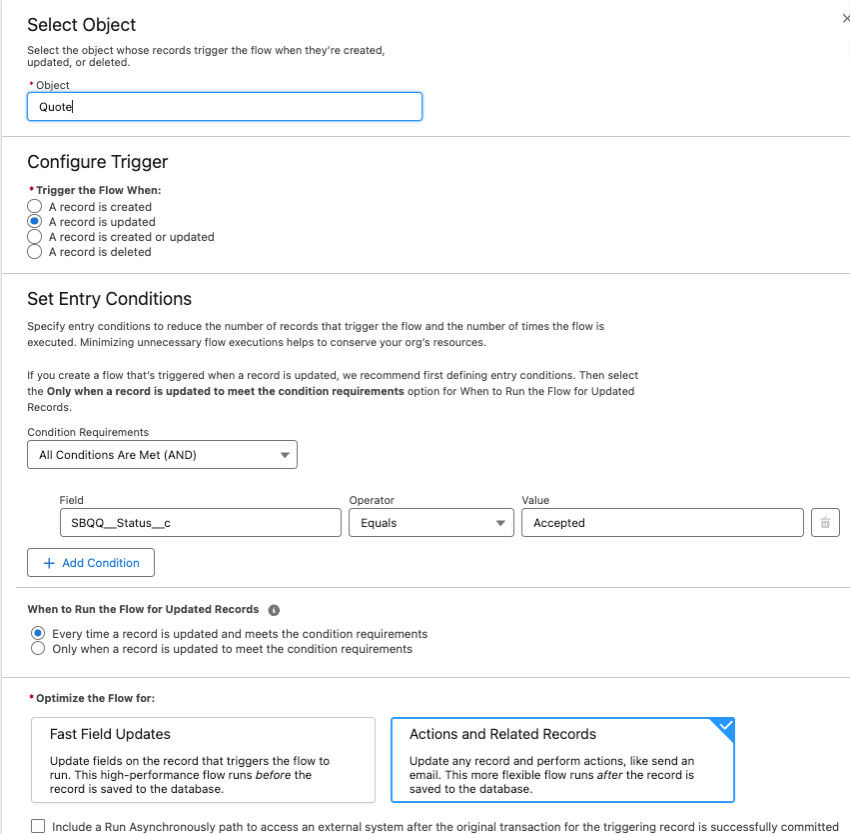
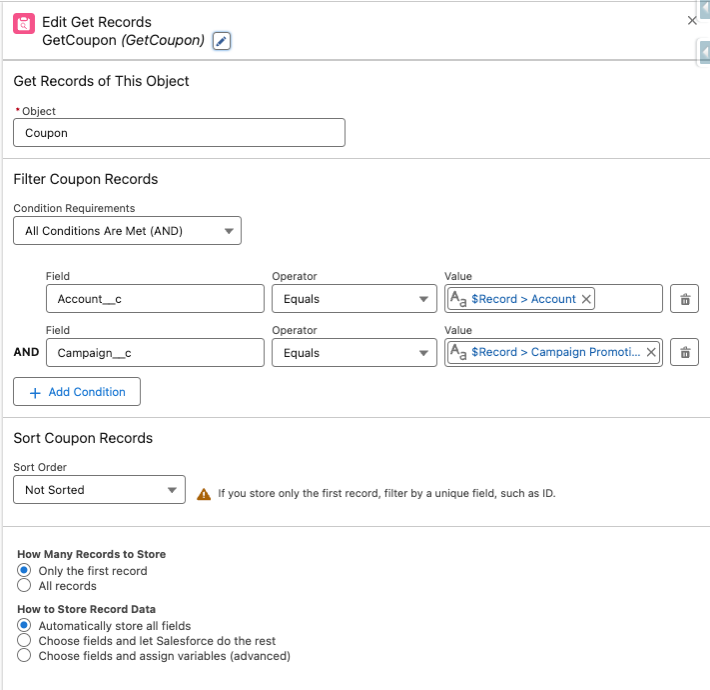
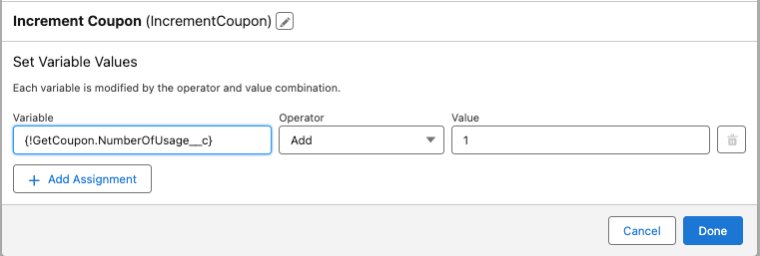
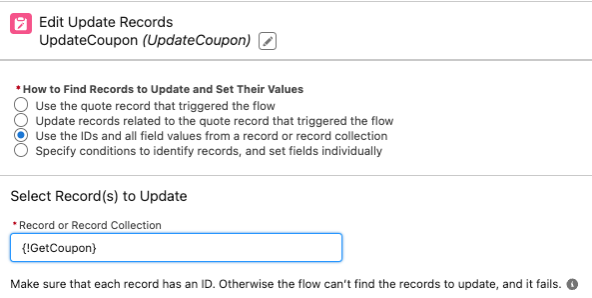
Note:
This part will count the number of usages for a campaign per account when the quote is accepted. To be strict, you can add a validation rule in quote in order to block any update from the quote and continue to count this quote as accepted.
CS
export function onBeforeCalculate(quoteModel, quoteLineModels, conn) {
return CouponLimit(quoteModel, quoteLineModels, conn); // Return the Promise from CouponLimit
};
// Function to set custom discounts based on the quote lines.
async function CouponLimit(quoteModel, _quoteLineModels, conn) {
console.log('quoteModel:', quoteModel);
console.log('quoteModel.record:', quoteModel.record);
console.log('CampaignPromotion__c:', quoteModel.record ? quoteModel.record["CampaignPromotion__c"] : 'record is undefined');
if (quoteModel.record["CampaignPromotion__c"]) {
const CampaignPromotionId = quoteModel.record["CampaignPromotion__c"];
try {
const resultsCoupon = await conn.query("SELECT Id, Account__c, Campaign__c, Name, NumberOfUsage__c FROM Coupon__c WHERE Campaign__c = '" + CampaignPromotionId + "'");
if (resultsCoupon) {
console.log('resultsCoupon:', resultsCoupon);
console.log('resultsCoupon.record:', resultsCoupon.records[0]);
console.log('resultsCoupon.record["NumberOfUsage__c"]', resultsCoupon.records[0] ? resultsCoupon.records[0]["NumberOfUsage__c"] : 'record is undefined');
const resultsCampaign = await conn.query("SELECT Id, Active__c, LimitUsage__c FROM Campaign__c WHERE Id = '" + CampaignPromotionId + "'");
if (resultsCampaign) {
console.log('resultsCampaign:', resultsCampaign);
console.log('resultsCampaign.record:', resultsCampaign.records[0]);
console.log('resultsCampaign.record["LimitUsage__c"]', resultsCampaign.records[0] ? resultsCampaign.records[0]["LimitUsage__c"] : 'record is undefined');
const couponUsage = resultsCoupon.records[0].NumberOfUsage__c;
const limitUsage = resultsCampaign.records[0].LimitUsage__c;
console.log("coupon usage" + couponUsage)
console.log("limit Usage" + limitUsage)
if (couponUsage >= limitUsage) {
alert('Error: Coupon usage limit exceeded.');
quoteModel.record["CampaignPromotion__c"]=null;
}
}
}
} catch (error) {
console.error('Error in CouponLimit function:', error);
// Handle any other errors, possibly showing another alert or handling it differently
}
}
return Promise.resolve();
}
Conclusion
Now with this you can block the campaign if it exceeds the limit of usage
Result
the link for the github: https://github.com/AourLegacy/AourFactory/tree/Coupon-limit
Part 2
Introduction
I want to apply all the campaign which doesn’t have code. When I want to add a campaign code, I need to check if this is code is cumulable with the code campaign doesn’t have code, how to do it?
Object you need:
Campaign
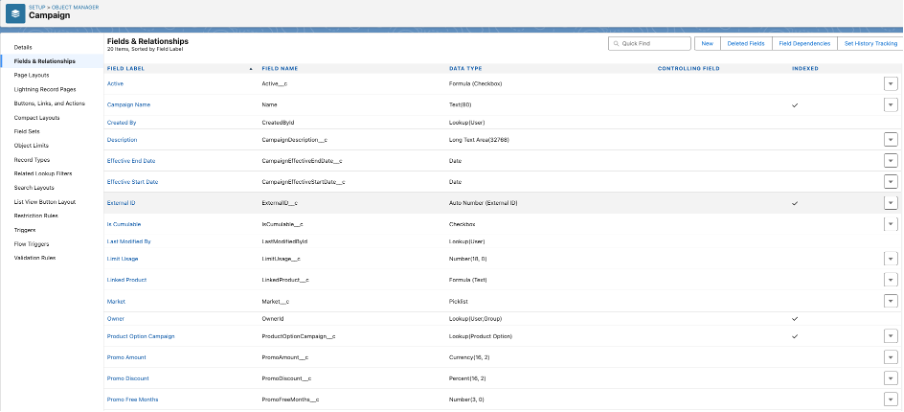

Field you need:
In quote:
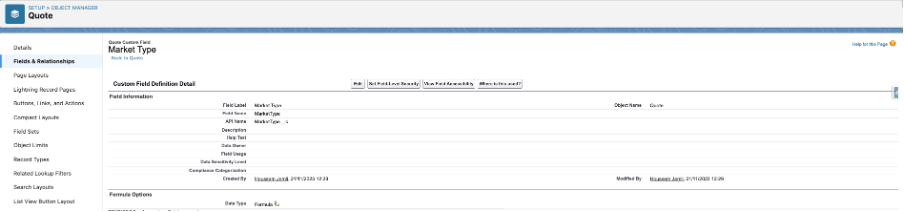
Custom script
// Function triggered after the calculation step in a quote.
export function onAfterCalculate(quoteModel, quoteLineModels, conn) {
// Call the function to apply cumulable coupons, if applicable.
CumulableCoupon(quoteModel, quoteLineModels, conn);
// Returns a resolved promise as this is the end of the operation.
return Promise.resolve();
};
// Asynchronous function to handle the application of cumulable coupons.
async function CumulableCoupon(quoteModel, quoteLineModels, conn) {
var AmountWithCodePromo=0
var PercentageWithCodePromo=0
var AmountWithoutCodePromo=0
var PercentageWithoutCodePromo=0
quoteModel.record.SBQQ__TargetCustomerAmount__c=null
quoteModel.record.SBQQ__CustomerDiscount__c=null
// Extract the market account information from the quote.
var MarketAccount = quoteModel.record.MarketType__c;
// Check if a campaign code is present in the quote.
if (quoteModel.record['CampaignCode__c']) {
// Query the Campaign__c object for active campaigns matching the market account and the provided campaign code.
var resultsCampaignWithPromoCode = await conn.query("SELECT Id, Active__c, Market__c, Promocode__c, PromotionType__c, PromoAmount__c, PromoDiscount__c, IsCumulable__c FROM Campaign__c WHERE Active__c = True AND Market__c='" + MarketAccount + "' AND Promocode__c='" + quoteModel.record['CampaignCode__c'] + "'");
// Logging the results of the query for debugging.
if (resultsCampaignWithPromoCode.totalSize==1) {
// Check if the campaign is cumulable and apply the appropriate discounts.
if (resultsCampaignWithPromoCode.records[0].IsCumulable__c == true) {
// Apply an amount discount if the promotion type is 'Amount'.
if (resultsCampaignWithPromoCode.records[0].PromotionType__c == 'Amount') {
AmountWithCodePromo = resultsCampaignWithPromoCode.records[0].PromoAmount__c;
}
// Apply a percentage discount if the promotion type is 'Percentage'.
if (resultsCampaignWithPromoCode.records[0].PromotionType__c == 'Percentage') {
PercentageWithCodePromo = resultsCampaignWithPromoCode.records[0].PromoDiscount__c;
console.log("PercentageWithCodePromo "+ PercentageWithCodePromo)
}
}
}
}
// Query the Campaign__c object for active campaigns in the market account without a specific promo code.
var resultsCampaignWithoutPromoCode = await conn.query("SELECT Id, Active__c, Market__c, Promocode__c, PromotionType__c, PromoAmount__c, PromoDiscount__c FROM Campaign__c WHERE Active__c = True AND Market__c='" + MarketAccount + "' AND Promocode__c=''");
// Loop through the results and apply applicable promotions.
if (resultsCampaignWithoutPromoCode) {
if (resultsCampaignWithoutPromoCode.totalSize > 0) {
resultsCampaignWithoutPromoCode.records.forEach(Promo => {
// Apply an amount discount if the promotion type is 'Amount'.
if (Promo.PromotionType__c == 'Amount') {
AmountWithoutCodePromo = Promo.PromoAmount__c;
}
// Apply a percentage discount if the promotion type is 'Percentage'.
if (Promo.PromotionType__c == 'Percentage') {
PercentageWithoutCodePromo = Promo.PromoDiscount__c;
console.log("PercentageWithoutCodePromo "+ PercentageWithoutCodePromo)
}
});
}
}
quoteModel.record.SBQQ__TargetCustomerAmount__c = quoteModel.record.SBQQ__ListAmount__c - (AmountWithCodePromo+AmountWithoutCodePromo)
quoteModel.record.SBQQ__CustomerDiscount__c= PercentageWithCodePromo + PercentageWithoutCodePromo
}
Conclusion
In the case you apply the promotion on the quote, the additional discount seems to not working if you fill this field. Maybe, there is an another to fill it.
Result
the link for the github : https://github.com/AourLegacy/AourFactory/tree/Cumulable-Coupon
Comments